With the Magic Light Bulb circuit built on the MakerShield, it’s time to upload the sketch. Example 10-1 operates the RGB LEDs using a mini pushbutton switch. Here are the steps you’ll need to follow:
- Attach the Arduino to your computer using a USB cable.
- Open the Arduino software and type Example 10-1 into the software’s text editor.
- Upload the sketch to the Arduino.
- Press the mini pushbutton switch for a moment.
The Arduino will sequence the RGB LED tricolor pattern three times. Figure 10-3 shows the Magic Light Bulb in action.
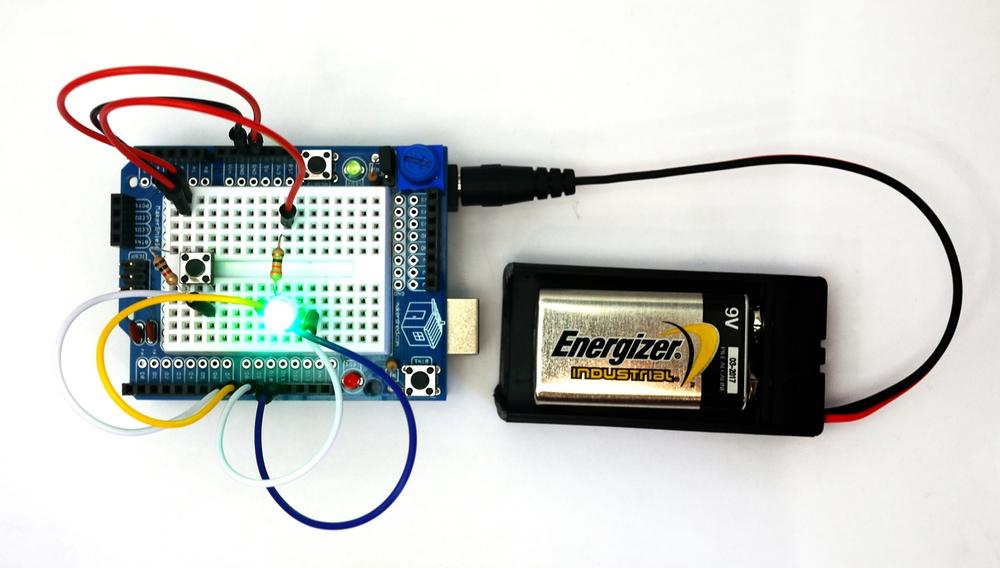
Figure 10-3. The Magic Light Bulb running through the tricolor pattern
Example 10-1. The Magic Light Bulb sketch
/*
Magic Light Bulb
Flashes the red, green, and blue LEDs of an RGB LED three times by
briefly pressing a mini pushbutton switch.
25 Feb 2013
Don Wilcher
*/
// Pushbutton switch and RGB pins wired to the Arduino microcontroller.
// give them names:
int redled = 9;
int grnled = 10;
int bluled = 11;
int Pbutton = 8;
// initialize counter variable
int n =0;
// monitor pushbutton switch status:
int Pbuttonstatus = 0;
// the setup routine runs once when you press reset:
void setup() {
// initialize the digital pins as outputs:
pinMode(redled, OUTPUT);
pinMode(grnled, OUTPUT);
pinMode(bluled, OUTPUT);
// initialize the digital pin as an input:
pinMode(Pbutton, INPUT);
// turn RGB outputs off:
digitalWrite(redled, HIGH);
digitalWrite(grnled, HIGH);
digitalWrite(bluled, HIGH);
}
// the loop routine runs 3x after the pushbutton is pressed:
void loop() {
Pbuttonstatus = digitalRead(Pbutton); // read pushbutton status
if(Pbuttonstatus == HIGH) { // if it's HIGH, start RGB Flasher
for (n = 0; n < 3; n++){ // flash RGB LEDs 3x
digitalWrite(redled, LOW); // turn the red LED on (LOW is on)
delay(250); // wait for a 1/4 second
digitalWrite(redled, HIGH); // turn the LED off (HIGH is off)
delay(250); // wait for a 1/4 second
digitalWrite(grnled, LOW); // turn the green LED on
delay(250); // wait for a 1/4 second
digitalWrite(grnled, HIGH); // turn the green LED off
delay(250); // wait for a 1/4 second
digitalWrite(bluled, LOW); // turn the blue LED on
delay(250); // wait for a 1/4 second
digitalWrite(bluled, HIGH); // turn the blue LED off
delay(250); // wait for a 1/4 second
}
}
else{ // if pushbutton is LOW, turn LEDs off
digitalWrite(redled, HIGH);
digitalWrite(grnled, HIGH);
digitalWrite(bluled, HIGH);
}
}
TROUBLESHOOTING TIP
If the LEDs don’t turn on in the proper sequence, check your sketch pin assignments as well as the orientation of the component on the MakerShield mini breadboard.
THE FOR LOOP AND COUNTERS
When you briefly press the mini pushbutton switch, the RGB cycles its color sequence three times before turning off. A “for” loop is behind the magic. Here’s the Arduino sketch code:
for (n = 0; n < 3; n++)
How does it work? The counter starts at zero (n = 0
) and checks to see if the count value is less than 3 (n < 3
). If the counter is less than 3, the counter adds one to itself (n++
) to get the next count value. When the counter reaches a value of 3, the Arduino turns off the RGB LED.
Change the “3” to a “4” in the preceding code, and observe the RGB LED after uploading the modified sketch to the Arduino. Remember to record your observations and modified sketches into your lab notebook!
Leave a Reply