With the Arduino AND Logic Gate built on the MakerShield, it is time to upload the sketch. Example 6-1 operates the green LED using a pushbutton switch and a photocell. Here are the steps you’ll need to follow:
- Attach the Arduino to your computer using a USB cable.
- Open the Arduino software and type Example 6-1 into the software’s text editor.
- Upload the sketch to the Arduino.
- Press the mini pushbutton switch for a moment.
The Arduino AND Logic Gate will turn on the LED when the photocell is covered and the pushbutton switch is pressed. Releasing the pushbutton switch, or placing a light on the photocell, turns the LED off, because the AND condition (in which both switches are closed) no longer exists.
The Arduino does this by using the &&
operator in the if statement. &&
is the computer programming symbol for the logical AND function.
Example 6-1. The Arduino AND Logic Gate sketch
/*
The Arduino AND Logic Gate
Turns on an LED connected to digital
pin 7, when pressing a pushbutton switch and covering a photocell
attached to pins 3 and 4.
27 Jan 2013
Revised 4 September 2013
by Don Wilcher
*/
// constants won't change; they're used here to
// set pin numbers:
int B = 3; // the number of the B pushbutton pin
int A = 4; // the number of the A pushbutton pin
const int Cout = 7; // the number of the LED pin
// variables will change:
int AStatus = 0; // variable for reading the A pushbutton status
int BStatus = 0;
void setup() {
// initialize the LED pin as an output:
pinMode(Cout, OUTPUT);
// initialize the pushbutton pins as inputs:
pinMode(B, INPUT);
pinMode(A, INPUT);
}
void loop(){
// read the state of the pushbutton value:
AStatus = digitalRead(A);
BStatus = digitalRead(B);
// check if the pushbuttons are pressed
// if it is, the buttonStatus is HIGH:
if (AStatus == HIGH && BStatus ==HIGH) {
// turn LED on:
digitalWrite(Cout, HIGH);
}
else {
// turn LED off:
digitalWrite(Cout, LOW);
}
}
After uploading the Arduino AND Gate Logic sketch to the Arduino microcontroller, the green LED is turned off. As discussed earlier, pressing the pushbutton switch and covering the photocell will turn on the green LED. If either input device is FALSE (logic LOW), the LED turns off. To completely test the Arduino AND Logic Gate’s operation, remember to use the TT shown in Figure 6-5.
The block diagram in Figure 6-9 shows the building blocks and the electrical signal flow for the Arduino AND Logic Gate. Circuit schematic diagrams are used by electrical engineers to quickly build cool electronic devices. The equivalent circuit schematic diagram for the Arduino AND Logic Gate is shown in Figure 6-10.
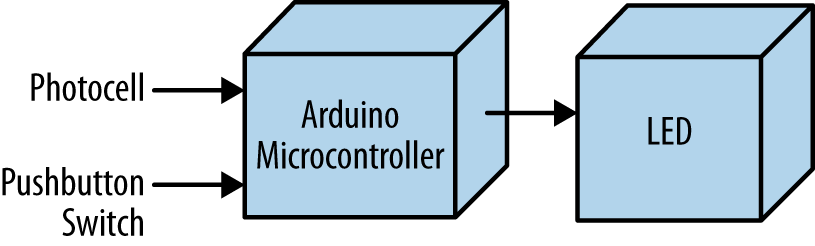
Figure 6-9. The Arduino AND Logic Gate block diagram
TROUBLESHOOTING TIP
If you don’t see the green LED turn on with the proper input logic, recheck the logic gate’s electrical wiring. Also, check to see if the green LED’s negative pin is wired to GND on the breadboard.
Leave a Reply