Before diving into the best practices, let’s get familiar with the mechanics of writing such tests. For that, we will rely on Selenium. The Selenium framework is a well-known tool that supports developers in testing web applications. Selenium can connect to any browser and control it. Then, through the Selenium API, we can give commands such as “open this URL,” “find this HTML element in the page and get its inner text,” and “click that button.” We will use commands like these to test our web applications.
We use the Spring PetClinic web application as an example throughout this section. If you are a Java web developer, you are probably familiar with Spring Boot. For those who are not, Spring Boot is the state-of-the-art framework for web development in Java. Spring PetClinic is a simple web application that illustrates how powerful and easy to use Spring Boot is. Its code base contains the two lines required for you to download (via Git) and run (via Maven) the web application. Once you do, you should be able to visit your localhost:8080 and see the web application, shown in figures 9.3 and 9.4.
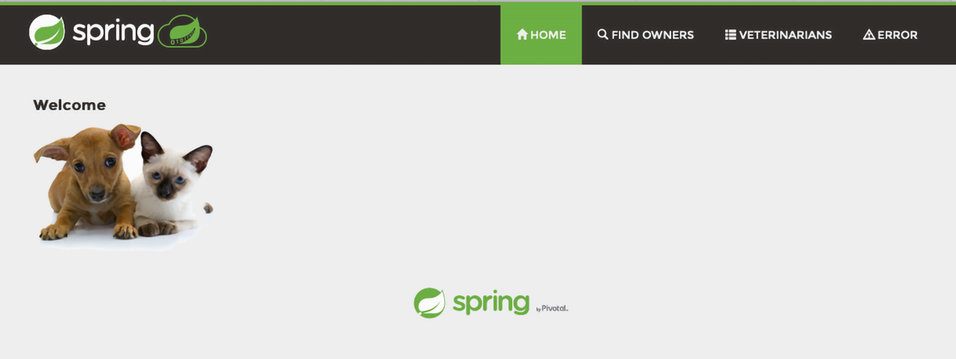
Figure 9.3 First screenshot of the Spring PetClinic application
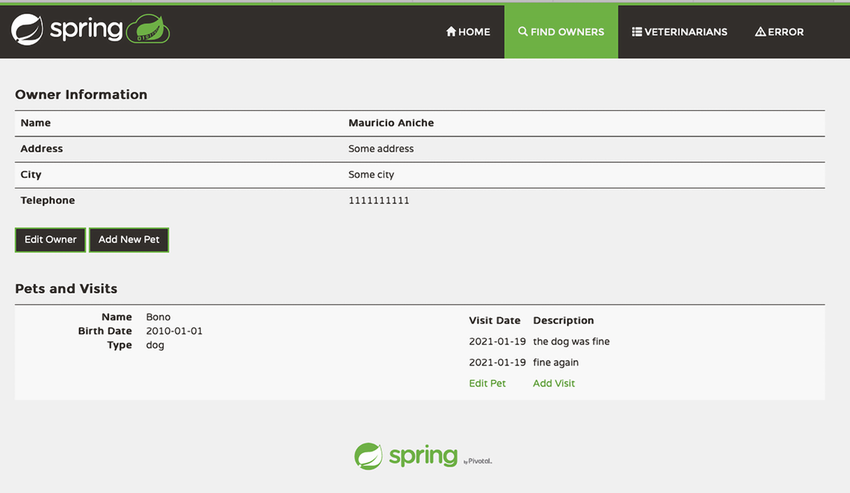
Figure 9.4 Second screenshot of the Spring PetClinic application
Before discussing testing techniques and best practices, let’s get started with Selenium. The Selenium API is intuitive and easy to use. The following listing shows our first test.
Listing 9.24 Our first Selenium test
public class FirstSeleniumTest {
@Test
void firstSeleniumTest() {
WebDriver browser = new SafariDriver(); ❶
browser.get("http:/ /localhost:8080"); ❷
WebElement welcomeHeader = browser.findElement(By.tagName("h2")); ❸
assertThat(welcomeHeader.getText())
.isEqualTo("Welcome"); ❹
browser.close(); ❺
}
}
❶ Selects a driver. The driver indicates which browser to use.
❷ Visits a page at the given URL
❸ Finds an HTML element in the page
❹ Asserts that the page contains what we want
❺ Closes the browser and the selenium session
- The first line,
WebDriver
browser
=
new
SafariDriver()
, instantiates a Safari browser.WebDriver
is the abstraction that all other browsers implement. If you would like to try a different browser, you can usenew
FirefoxBrowser()
ornew
ChromeBrowser()
instead. I am using Safari for two reasons:a) I am a Mac user, and Safari is often my browser of choice.b) Other browsers, such as Chrome, may require you to download an external application that enables Safari to communicate with it. In the case of Chrome, you need to download ChromeDriver. - With an instantiated browser, we visit a URL by means of
browser.get("url");
. Whatever URL we pass, the browser will visit. Remember that Selenium is not simulating the browser: it is using the real browser. - The test visits the home page of the Spring PetClinic web app (figure 9.3). This website is very simple and shows a brief message (“Welcome”) and a cute picture of a dog and a cat. To ensure that we can extract data from the page we are visiting, let’s ensure that the “Welcome” message is on the screen. To do that, we first must locate the element that contains the message. Knowledge of HTML and DOM is required here.If you inspect the HTML of the Spring PetClinic, you see that the message is within an
h2
tag. Later, we discuss the best ways to locate elements on the page; but for now, we locate the onlyh2
element. To do so, we use Selenium’sfindElement()
function, which receives a strategy that Selenium will use to find the element. We can find elements by their names, IDs, CSS classes, and tag name.By.tagName("h2")
returns aWebElement
, an abstraction representing an element on the web page. - We extract some properties of this element: in particular, the text inside the
h2
tag. For that, we call thegetText()
method. Because we expect it to return “Welcome”, we write an assertion the same way we are used to. Remember, this is an automated test. If the web element does not contain “Welcome”, the test will fail. - We close the browser. This is an important step, as it disconnects Selenium from the browser. It is always a good practice to close any resources you use in your tests.
If you run the test, you should see Safari (or your browser of choice) open, be automatically controlled by Selenium, and then close. This will get more exciting when we start to fill out forms.
Leave a Reply