After building the Rocket Game pushbutton circuit and checking for wiring errors, it is time to upload the sketch. Example 19-1 sends digital information to the Arduino IDE (integrated development environment) Serial Monitor, displaying the numbers 0, 1, 2, and 4 with each individual press of the four pushbutton switches. The serial communication technique used in remains the same for the Arduino software to talk with the Processing programming language. Here are the steps you’ll need to follow:
- Attach the Arduino to your computer using a USB cable.
- Open the Arduino software and type Example 19-1 into the software’s text editor.
- Upload the sketch to the Arduino.
The Serial Monitor will start to display numbers, as shown in Figure 19-3, each time you press the pushbutton. Pushing various combinations of switches will show new results. This information will be displayed in Processing, and will create an awesome visual of rockets being launched on the screen.
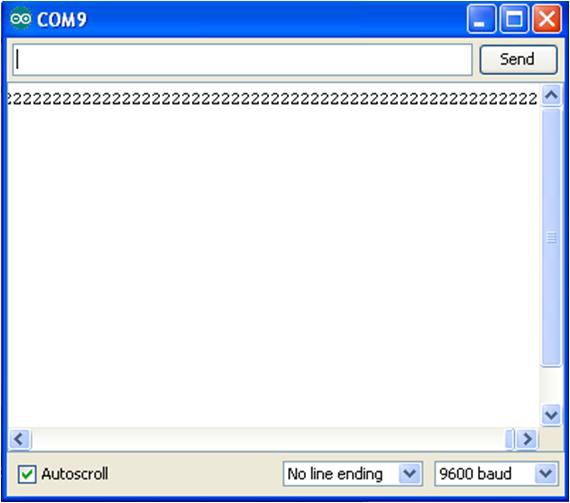
Figure 19-3. Decimal equivalent numbers being displayed on the Arduino Serial Monitor; pushbutton 3 has been pressed
0, 1, 2, AND … 4?
Why are the pushbuttons producing the numbers 0, 1, 2, and 4? What happened to 3? The answer is that the buttons have been programmed to count in binary, a number system that is based on the powers of 2. Zero is just that, zero. Two raised to the 0th power equals 1. Two raised to the 1st power equals 2. Two raised to the 2nd power equals 4.
Why the preoccupation with powers of two? As computers have two primary states (+5 volts and 0 volts), it’s easy to use those two states as the internal basis for everything the computer does. When computers need to count, add, or divide, they break the operation down into powers of two. In this project, instead of making the Arduino do the conversion, we’ve just started out using the powers of two.
Example 19-1. The MultiDigital4 sketch
/*
* MultiDigital4
*
* Reads 8 digital inputs and sends their values over the serial port.
* A byte variable is used to store the state of all eight pins. This byte
* is then sent over the serial port.
*
* modified ap_ReadDigital8 sketch by Melvin Oschmann
*
* 8 June 2013
* Don Wilcher
*
*/
// 8 variables for each pin
int digitalInput_1 = 3;
int digitalInput_2 = 4;
int digitalInput_3 = 5;
int digitalInput_4 = 6;
int digitalInput_5 = 7;
int digitalInput_6 = 8;
int digitalInput_7 = 9;
int digitalInput_8 = 10;
// 8 variables to store the values
int value_1 = 0;
int value_2 = 0;
int value_3 = 0;
int value_4 = 0;
int value_5 = 0;
int value_6 = 0;
int value_7 = 0;
int value_8 = 0;
// byte variable to send state of all pins over serial port
int myByte = 0;
// control LED
int controlLED = 13;
void setup(){
// set pin modes
pinMode(digitalInput_1, INPUT); pinMode(digitalInput_2, INPUT);
pinMode(digitalInput_3, INPUT); pinMode(digitalInput_4, INPUT);
pinMode(digitalInput_5, INPUT); pinMode(digitalInput_6, INPUT);
pinMode(digitalInput_7, INPUT); pinMode(digitalInput_8, INPUT);
pinMode(controlLED, OUTPUT);
// begin sending out over the serial port
Serial.begin(9600);
}
void loop(){
// set 'myByte' to zero
myByte = 0;
// then read all the INPUTS and store values
// in the corresponding variables
value_1 = digitalRead(digitalInput_1);
value_2 = digitalRead(digitalInput_2);
value_3 = digitalRead(digitalInput_3);
value_4 = digitalRead(digitalInput_4);
value_5 = digitalRead(digitalInput_5);
value_6 = digitalRead(digitalInput_6);
value_7 = digitalRead(digitalInput_7);
value_8 = digitalRead(digitalInput_8);
/* check if values are high or low and 'add' each value to myByte
* what it actually does is this:
*
* 00 00 00 00 ('myByte set to zero')
* | 00 10 10 00 ('3 and 5 are 1')
* --------------
* 00 10 10 00 ('myByte after logical operation')
*
*/
if (value_1) {
myByte = myByte | 0;
digitalWrite(controlLED, HIGH);
} else digitalWrite(controlLED, LOW);
if (value_2) { myByte = myByte | 1; }
if (value_3) { myByte = myByte | 2; }
if (value_4) { myByte = myByte | 4; }
if (value_5) { myByte = myByte | 8; }
if (value_6) { myByte = myByte | 16; }
if (value_7) { myByte = myByte | 32; }
if (value_8) { myByte = myByte | 64; }
// send myByte out over serial port and wait a bit to not overload the port
Serial.print(myByte);
delay(10);
}
Leave a Reply